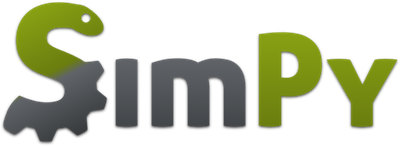
Discrete event simulation for Python
PyPI | GitLab | Issues | Mailing list
Overview
SimPy is a process-based discrete-event simulation framework based on standard Python.
Processes in SimPy are defined by Python generator functions and may, for example, be used to model active components like customers, vehicles or agents. SimPy also provides various types of shared resources to model limited capacity congestion points (like servers, checkout counters and tunnels).
Simulations can be performed “as fast as possible”, in real time (wall clock time) or by manually stepping through the events.
Though it is theoretically possible to do continuous simulations with SimPy, it has no features that help you with that. On the other hand, SimPy is overkill for simulations with a fixed step size where your processes don’t interact with each other or with shared resources.
A short example simulating two clocks ticking in different time intervals looks like this:
>>> import simpy
>>>
>>> def clock(env, name, tick):
... while True:
... print(name, env.now)
... yield env.timeout(tick)
...
>>> env = simpy.Environment()
>>> env.process(clock(env, 'fast', 0.5))
<Process(clock) object at 0x...>
>>> env.process(clock(env, 'slow', 1))
<Process(clock) object at 0x...>
>>> env.run(until=2)
fast 0
slow 0
fast 0.5
slow 1
fast 1.0
fast 1.5
The documentation contains a tutorial, several guides explaining key concepts, a number of examples and the API reference.
SimPy is released under the MIT License. Simulation model developers are encouraged to share their SimPy modeling techniques with the SimPy community. Please post a message to the SimPy mailing list.
There is an introductory talk that explains SimPy’s concepts and provides some examples: watch the video or get the slides.
SimPy has also been reimplemented in other programming languages. See the list of ports for more details.